Getting Started
On this page
This tutorial will show you how to add the Prysm plugin into a project and create two types of UI elements - a HUD
and an in-world UI View. Here are the steps to achieve this:
Setup
1. Install the Prysm plugin
First, you need to install the Prysm plugin using the installer wizard. The installer auto-detects the Unreal Engine versions that are available on a given computer and provides you with the options to install a sample game.
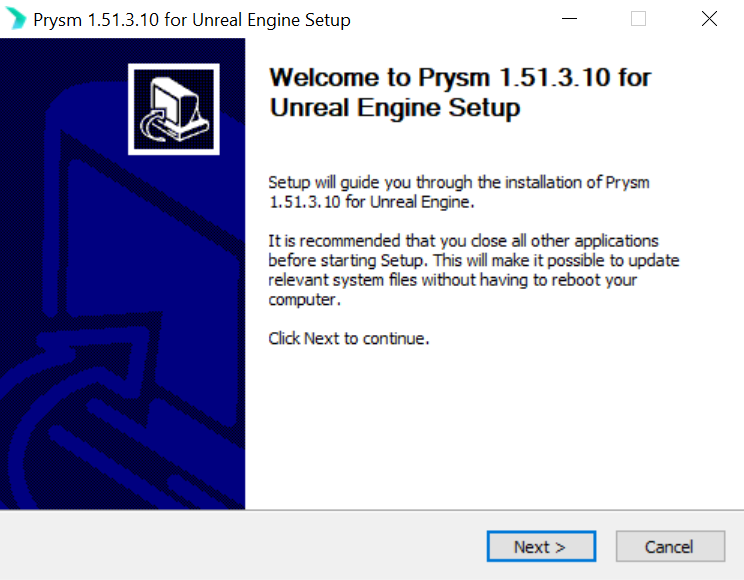
2. Configure the plugin in your project
Afterwards, start the Unreal Editor, and choose either an existing project, or create a new one via New Project -> Blueprint -> Blank, and save it in a directory of your choice.
Then create a new level and open the world settings. From the Game Mode section, click the + button to create a new Game Mode
override, let’s name it MyGameMode
.
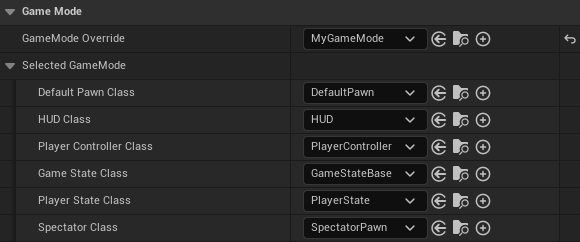
Now, from the main menu choose Edit -> Project Settings, and navigate to Maps & Modes in the Project section, then set the Default GameMode to MyGameMode
.
3. Add a HUD
From the Prysm menu, choose Add HUD. This will change the current GameMode’s HUD
class and will initialize it. Also, it will add a hud.html
page in YourProjectDir/Content/uiresources/
directory (if not already present), then this page will be opened in your default browser.
For the HUD
, you can add your UI resources in the YourProjectDir/Content/uiresources
directory, respectively. As we previously mentioned, the HUD
has been pre-setup for you and is ready to use, so you don’t need to do any modifications to it, except for changing the View
Page URL to your own HTML page like so coui://uiresources/YourHUD.html
.
Here’s a brief explanation of what happens in your new HUD
class:
- Setting up the
View
- the HTML page to load and otherView
-specific options - The UI input is initialized using a helper Blueprint function. You can double-click it to inspect it in more detail, but it basically involves spawning and initializing a
PrysmInputActor
, and then callingAlways Accept Mouse Input
and setting it totrue
.
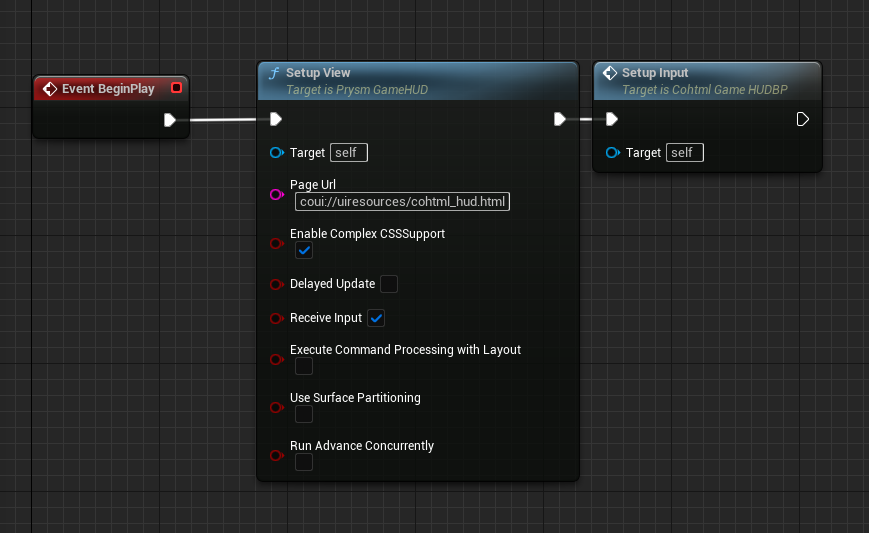
PrysmInputActor
will consume the input of any UMG Widgets you add to the viewport, since it covers the entire screen. If you prefer using UMG, you can find out how to do that here.The page is set to coui://uiresources/hud.html
by default, so the newly created page by Add HUD will be used if you skip changing it. However, you can change it whenever you want to a local page, placed in the uiresources
folder and use the coui://
scheme.
4. Add in-world UI
Choose Prysm -> Add In-world UI. This will add a plane to your scene, just in front of the camera. You can change its transform via the World Transform in the Details tab.
To change what UI is loaded, just change the URL property in the Details panel.
Here is the final result:
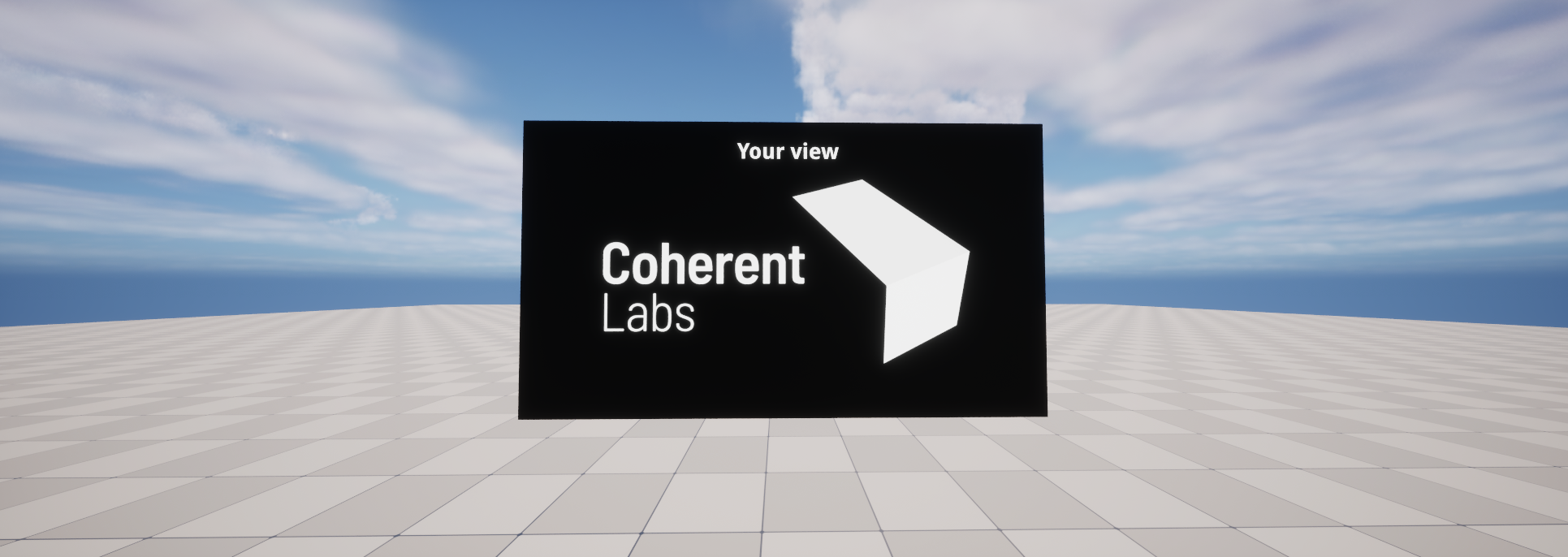
CohtmlMaterial
, which is not affected by scene lights. In case you want to have an in-world View that is affected by scene lights, you just need to change the Static Mesh Material of the CohtmlPlane
to CohtmlMaterialLit
. Both Materials will still be affected by any post-processing in the scene, including light effects done via the post-processing volume.Difference between Unlit and Lit Material for in-world Views
In order to showcase the difference between CohtmlMaterial and CohtmlMaterialLit Materials let’s compare them side by side with the same UI. In order to make it clearly visible, we can also add bright point lights that are also color tinted.
On hitting the Play
button in the Editor, the in-world View using the CohtmlMaterial remains unaffected by the lighting, while the one using CohtmlMaterialLit is properly lit and tinted.
MobaInGame.html
page, located under Content/uiresources/Moba
.Engine plugin or a Game plugin? Which one do I need?
Prysm can be installed as either Engine plugin or a Game plugin. Each of them having their own merit.
Engine plugin
This is the default option of installation. When you have Prysm installed as an Engine plugin, the plugin will be available for all of the games that are created with that particular instance of Unreal Engine. This is useful when you are working on more than one game at the same time, or you are prototyping.
Game plugin
Usually the Game plugin option is used when using a binary engine. This is because this is the only way to build a plugin when using a binary engine. Another reason why you would like to use Prysm as a Game plugin would be if you don’t want the plugin to be visible to other games using the same engine.
Plugin structure
Prysm’s plugin uses three modules:
- One for the actual Cohtml C++ shared libraries (
.dll
,.dylib
, etc.); - An Engine module that sets up the dependency to the core Cohtml library. It exposes several classes and Components specific for the development with Unreal Engine and takes care of all the initialization and management of Cohtml.
- An Editor module which eases your workflow with Prysm in the Unreal Editor.
Prysm also has one plugin as a dependency - CoherentRenderingPlugin, which in turn consists of two modules:
- One for the actual Renoir C++ shared libraries (
.dll
,.dylib
, etc.); - An Engine module that sets up the dependency to the core Renoir library. It takes care of all the initialization and management of the Renoir rendering library and contains the rendering backend which serves as the glue between the library and Unreal’s RHI.
Setting up the ThirdParty dependency
Prysm has to be added as a third-party dependency to Unreal. This will make sure that all shared libraries (.dll
, .dylib
, etc.) are loaded when the game starts and they will be referenced by the engine correctly.
Install steps:
- Run the provided installer.
- When asked what to install, check UnrealEngine Plugin.
- Select which Unreal Engine version you’d like to install the plugin to. 3.1 If you’ve selected a binary distribution, Prysm will be installed as a Game plugin, and you will be asked for the path to the game. 3.2 If you’ve selected an compiled from source distribution, Prysm will be installed as an Engine plugin.
- Click install.
The installer provides some other useful items, such as a sample game and a collection of ready-to-use HTML5 kits.
Add Prysm to your game
If you are only going to use Prysm through the Unreal Editor / Blueprints, you only need to enable the plugin. If you are going to use Prysm through C++ code, make sure your game references our plugin in its Build.cs
file.
Doing so is trivial - open YourGame/Source/YourGame/YourGame.Build.cs
and add the following lines to the constructor of the class YourGame
:
PrivateDependencyModuleNames.Add("CohtmlPlugin");
PrivateDependencyModuleNames.Add("Cohtml");
.uproject
file of your game."Plugins": [
{
"Name": "CohtmlPlugin",
"Enabled": true
}
]
coui://
) in YourGame/Content
folder.CohtmlPlugin.uplugin
and CoherentRenderingPlugin.uplugin
files from Runtime
to ClientOnly
. The reason for this change is to make sure that if you are packaging your game as a server, no Prysm-related code will run on that server. However, this introduces issues if you try to run the Unreal Editor itself as a server (using the -server
Command Line Argument), as the loading of Prysm-specific modules will happen too late and cause Unreal asserts to be triggered. In such cases, you will have to change the Module Type
of the .uplugin
files back to Runtime
, or disable the plugins altogether. Additionally, if in the Editor you try to play the Scene as a Standalone Game
with a Net Mode
set to Play As Client
, the server will not be started properly, because it will try to load the CohtmlSample
module and fail.You can find the plugin directories under the following path: GameOrEngineDir/Plugins/Runtime/Coherent
.
Plugin core classes
The plugin consists of several core classes. Below they are listed with their C++ names (UCohtml...
). You can find them by the name UPrysm...
in the Editor.
UCohtmlBaseComponent
(displayed asUPrysmBaseComponent
in the Editor) is an abstract class, actor component, which contains the necessary code to hook Cohtml up with Unreal Engine.UCohtmlComponent
is derived from theBase Component
and can be used to add a UI in the 3D world. It’s one of the core Prysm classes and represents a single HTML page that is rendered. Also holds methods for manipulating the View.UCohtmlHUD
is derived from theBase Component
and can be added to anAHUD
actor to addHUD
.ACohtmlGameHUD
is aHUD
class that is derived fromAHUD
and is the easiest way to use Prysm - you can just replace yourAHUD
class with this one. It’s a wrapper aroundUCohtmlHUD
with fairly simple implementation. This should be your main starting point if you want to have a Prysm View attached to your viewport.ACohtmlInputActor
is theInput Actor
responsible for capturing and forwarding mouse and keyboard input to the currently focused Prysm View. It must be spawned and initialized in the game world.SCohtmlWidget
is a Slate Widget capable of running Prysm.UCohtmlWidget
is an UMG Widget capable of running Prysm.
Adding the Prysm plugin to a Blueprint game
Using the installer
- Run the provided installer.
- When asked what to install, check Add Prysm to an existing game and uncheck Generate Project Files.
- Browse to the root directory of your game.
- Click install.
These are almost the same steps as in the previous section, but generating project files will fail if your game has no C++ code.
One last step
Open the Editor and enable the Prysm plugin for your game, using the Window -> Plugins dialog. Prysm plugin is in the Installed / User Interface section.
Prysm Components in the 3D world
Attaching a Prysm Component to a 3D object in the game world is actually a very easy thing to do - all you have to do is:
- Add a
UCohtmlComponent
to the object - In the Static Mesh Component of the object, change the material used to CohtmlMaterial.
Sample game
To quickly try out Prysm - try the provided CoherentSample game. In this project you will find the SampleHub
UE map (located in Content/Maps
) which is the entry point of our samples. The individual sample maps located in Content/Maps/SampleMaps
are using a mixture of C++ code and Blueprints, showcasing how the technology can be used in your UE game in different ways.
SampleHub
map you can click a sample of your choice to open. This will load a new map and you can press the “H” keyboard key to return to the entry map at any time.Prysm Launcher
The Prysm Launcher application will take you through some of the UI kits and samples we have created and give you an idea of what you can expect to find inside the CoherentSample game. Start the QuickStart.lnk / QuickStartMac.sh to open the Prysm Launcher application.
Preview files in the Cohtml Player
The Player application allows you to quickly preview any UI created for Prysm. You can find the Player application inside the CoherentSample game. Start the Player.bat / PlayerMac.sh inside the sample game to launch the Player application and drag and drop your HTML file.
TypeScript Declaration files
If you are using TypeScript, you can find the declaration files for Prysm inside GameOrEngineDir/Plugins/Runtime/Coherent/CohtmlPlugin/Resources
. For more information on how to import them, you can visit the native (custom engine) Prysm documentation.