Web Sockets
Prysm has built-in web socket support, which allows receiving data from a server without rerouting the connection through JavaScript Binding.
The Prysm API supports the functionality described in the MDN documentation. Prysm provides you with an example scene called “Websockets”. Import it from Prysm package from Unity3D package manager.
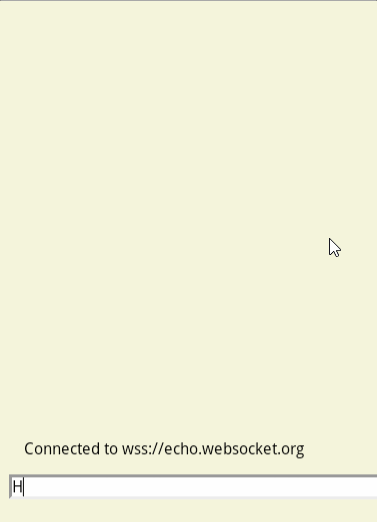
You can set the WebSocket client requests timeout from the CohtmlView.ViewListener
class through the OnWebsocketTimeoutSet
delegate. Call the method in your script’s Awake method. For example:
public class MyObject : MonoBehaviour
{
void Awake()
{
var view = GetComponent<CohtmlView>();
view.Listener.OnWebsocketTimeoutSet += () =>
{
return 3000; // timeout set to 3 seconds or 3000 milliseconds.
};
}
}
If you want to check whether the status of the WebSocket
is Open or Closed, you can use the cohtml.CohtmlView.ViewListener
Action property, which provides the WebSocketState
as an argument. For example:
public class MyObject : MonoBehaviour
{
void Awake()
{
CohtmlView view = GetComponent<CohtmlView>();
view.Listener.OnWebsocketStateChanged += state =>
{
Debug.Log($"State: {state}");
};
}
}